Some changes in .NET BCL 4.0
I’ve been porting a few products to .NET 4.0 and came across some cool new additions in .NET 4.0 which will be quite useful for developers.
Strings
Like the
String.IsNullOrEmpty
method, this new helper method will check whether the string is null, empty or contains only whitespace characters (fromChar.IsWhiteSpace
)Clear a StringBuilder contents instead of (the currently) setting of length = 0.
String.Join (string, params[])
Now you can easily join multiple strings without having to use an array.
Streams
Remember writing this before to copy one stream to another?
Now you don’t need to, just use the Stream.CopyTo()
method.
Checking for 64bit-ness
Previously to detect a 64bit operating system you would either P/Invoke out and call the IsWow64Process
in Kernel32, looked at the “PROCESSOR_ARCHITECTURE
” environment variable or even easier (and completely managed code) way of checking the size of a Pointer.
Now you can simply use the Environment
class that comes with two new properties.
Environment.Is64BitOperatingSystem()
– Are we running on a 64bit operating system?Environment.Is64BitProcess()
– Are we running as a 64bit process?
WPF 4.0 Improvements
There are simply too many to list, see the article on ScottGu‘s blog about WPF4 and VS2010/.NET 4.0.
One very important tweak are the Text Rendering improvements that TextBlock‘s now have a new TextOptions.TextFormattingMode that greatly improves the quality of text rendering.
Here’s a pretty picture showing the difference between using Ideal and Display. The difference is noticeable for text sizes below 15.
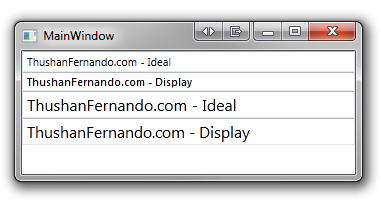
Alternatively you can place it in the Window so all child controls will render nicely.
There are LOTS more coming in .NET 4.0 that will make anyone doing .NET development today just wet their pants over, just read the article on MSDN by Justin Van Patten about Whats new in the BCL in .NET 4.0 and also posted on the BCL team blog.